In This Article
A step-by-step tutorial on how to convert list to tuple in Python, discussing different ways in which we can do the list tuple conversion.
In Python, lists and tuples are both utilized for storing collections of data, with a crucial distinction: lists are mutable, allowing modifications, while tuples are immutable, ensuring data integrity and safeguarding against unintended changes. This immutability makes tuples valuable in various scenarios.
Understanding the conversion from lists to tuples is pivotal in Python programming.
Why convert Python list to tuples?
Before we dive into the specifics to convert list to a tuple, note that even though lists and tuples are both sequences of items in Python.
They have some important differences.
- Lists are mutable, meaning that you can add, remove, or modify items within them.
- Tuples, on the other hand, are immutable, meaning that once they’re created, you can’t change them.
Consider a scenario where you have a list representing a set of constant values, and you want to ensure that these values remain unchanged throughout your program’s execution.
Converting list to a tuple in Python becomes essential to safeguard against inadvertent alterations that could compromise the integrity of the data.
So, now that we know why we need to convert from list to tuple, we will now investigate different ways to do so.
Different Ways How to convert List to Tuple in Python?
In the upcoming sections of this tutorial, we will delve into multiple methods in Python to convert lists to tuples.
Each method will be presented with Python code examples that will illustrate how that approach can be used. Then we can adapt the examples to our specific use case.
So, let’s begin with using the tuple
constructor.
Python Convert list to tuple using tuple constructor
The tuple constructor is a built-in function in Python that allows developers to create tuples from other iterable objects, such as lists.
While tuples can be defined explicitly using parentheses, the tuple
constructor can be used to transform existing collections.
The example code below illustrates the use of the tuple
constructor to create a list from a tuple:
my_list = [1, 2, 3, 4, 5]
my_tuple = tuple(my_list)
print(my_tuple)
In this example, the tuple
constructor is invoked with my_list
as an argument, generating a new tuple (my_tuple
) containing the same elements as the original list.
This one-liner encapsulates the entire process of list to tuple conversion, making the code concise and readable.
The tuple
constructor approach offers a direct and simple approach to convert lists to tuples. In the next section, we will consider using list unpacking to convert lists to tuples.
Convert list to tuples using * operator in Python
The *
operator, also known as the unpacking or Python spread operator, is a feature used for unpacking elements from iterable objects.
Using this Python operator in conjunction with parentheses can be used as a means to convert list to tuple.
Consider the following Python example for list to tuple conversion:
my_list = [1, 2, 3, 4, 5]
my_tuple = (*my_list,)
print(my_tuple)
The expression *my_list
unpacks the elements of my_list
, and the trailing comma within the parentheses ensures the creation of a tuple with those unpacked elements. This one line encapsulates the process to convert list to tuple elegantly.
Change list to tuple in Python Using generator expression
Generator expressions are a concise way to create iterators in Python.
Unlike list comprehensions that construct lists, generator expressions produce iterators on-the-fly, generating values one at a time rather than creating an entire sequence in memory.
This characteristic makes them well-suited for scenarios where memory efficiency and a dynamic approach are paramount.
The code for using a generator expression to convert a list into a tuple is succinct and expressive:
my_list = [1, 2, 3, 4, 5]
my_tuple = tuple(item for item in my_list)
print(my_tuple)
In this example, the generator expression (item for item in my_list)
is enclosed within the tuple
constructor, creating a tuple with elements derived from the original list.
The dynamic nature of generator expressions ensures that the elements are generated on-the-fly, making them memory-efficient, especially when dealing with large datasets.
All of these methods will convert list into tuple in Python, and you can choose the one that best fits your specific use case. However, we want to give a real example of how to convert a list into a tuple to put the concept into context.
Convert list to tuple for dictionary keys | Example
Suppose we have a dataset containing location information in the form of latitude, longitude, and place name.
We want to store this data in a dictionary where the key is the coordinates (latitude and longitude) and the value is the place name.
Below is a code illustrating how we can do it. However, the code will raise an exception:
import pprint
# Our dataset containing location information
data = [
[51.5074, -0.1278, "London"],
[40.7128, -74.0060, "New York City"],
[34.0522, -118.2437, "Los Angeles"],
[41.8781, -87.6298, "Chicago"],
[29.7604, -95.3698, "Houston"],
[33.4484, -112.0740, "Phoenix"],
[39.9526, -75.1652, "Philadelphia"],
[29.4241, -98.4936, "San Antonio"],
[32.7157, -117.1611, "San Diego"],
[37.7749, -122.4194, "San Francisco"],
[47.6062, -122.3321, "Seattle"],
[33.7490, -84.3880, "Atlanta"],
[35.2271, -80.8431, "Charlotte"],
[32.7767, -96.7970, "Dallas"],
[25.7617, -80.1918, "Miami"],
[40.4406, -79.9959, "Pittsburgh"],
[39.2904, -76.6122, "Baltimore"],
[38.9072, -77.0369, "Washington, D.C."],
[36.7783, -119.4179, "Fresno"],
[37.3382, -121.8863, "San Jose"]
]
# Extract the coordinates separately from the place names
coord_list = [e[:-1] for e in data]
place_list = [e[-1] for e in data]
# Initialize the final dict structure
dict_data = {}
# store the data into the dictionary in the form
# key: coordinates
# value: location name
for i in range(len(data)):
location = coord_list[i]
place = place_list[i]
# Add the location, place pair to the dictionary
dict_data[location] = place
# Display the final dictionary
pprint.pprint(dict_data)
In our code, we first extract the coordinates and place names from the original dataset into separate lists using list comprehension.
Then, within the loop, we try to add the key (location
) value (place
) pair into the dictionary dict_data
.
If we run this code, we will get the following error message:
Why did we get the error message?
When we added the location, place pair to the dict_data
dictionary, the value of location
used as a key is of type list
.
Python dictionaries use hashable types as dictionary keys, and lists are not hashable since they are mutable.
Solving the error by converting list to tuple in Python
In order to correct this bug, make sure each coordinate list is converted to a tuple.
We can use any of the previously explored techniques to do this.
See the next code listing for the corrected code:
import pprint
# Our dataset containing location information
data = [
[51.5074, -0.1278, "London"],
[40.7128, -74.0060, "New York City"],
[34.0522, -118.2437, "Los Angeles"],
[41.8781, -87.6298, "Chicago"],
[29.7604, -95.3698, "Houston"],
[33.4484, -112.0740, "Phoenix"],
[39.9526, -75.1652, "Philadelphia"],
[29.4241, -98.4936, "San Antonio"],
[32.7157, -117.1611, "San Diego"],
[37.7749, -122.4194, "San Francisco"],
[47.6062, -122.3321, "Seattle"],
[33.7490, -84.3880, "Atlanta"],
[35.2271, -80.8431, "Charlotte"],
[32.7767, -96.7970, "Dallas"],
[25.7617, -80.1918, "Miami"],
[40.4406, -79.9959, "Pittsburgh"],
[39.2904, -76.6122, "Baltimore"],
[38.9072, -77.0369, "Washington, D.C."],
[36.7783, -119.4179, "Fresno"],
[37.3382, -121.8863, "San Jose"]
]
# Extract the coordinates separately from the place names
coord_list = [e[:-1] for e in data]
place_list = [e[-1] for e in data]
# Initialize the final dict structure
dict_data = {}
# store the data into the dictionary in the form
# key: coordinates
# value: location name
for i in range(len(data)):
# Convert the coord_list[i] list into a tuple
location = tuple(coord_list[i])
place = place_list[i]
# Add the location, place pair to the dictionary
dict_data[location] = place
# Display the final dictionary
pprint.pprint(dict_data)
By converting the list of coordinates into a tuple, we have solved the problem of using a list (an unhashable type) as a key in a dictionary.
We get the following output:
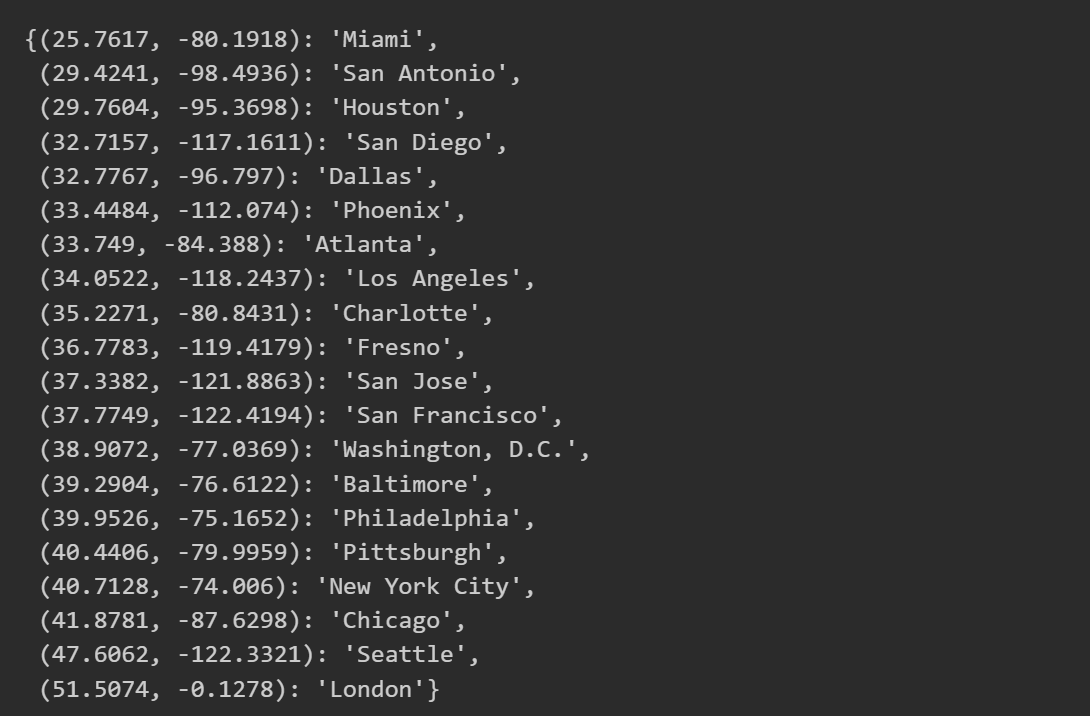
In conclusion, this tutorial explored various ways for convert list to tuple.
Three distinct methods were discussed, each illustrated with code examples: utilizing the tuple constructor, employing the *
operator for list unpacking, and leveraging generator expressions.
Additionally, a practical example involving geographical data emphasized the importance of using hashable types as dictionary keys, showcasing the significance of converting lists to tuples in real-world scenarios.
If you loved this tutorial, we have more Python tutorials in our doc section. Feel free to check them out.